What is Advanced Codings
Advanced coding is sophisticated programming techniques and methodologies to develop complex software systems. These techniques go beyond basic programming knowledge, including advanced algorithms, data structures, software design patterns, concurrent and parallel programming, and more. Here are some key areas in advanced coding:
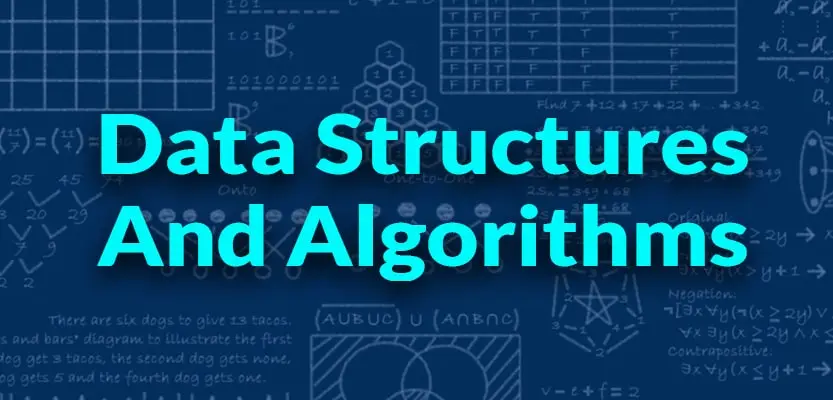
1. Advanced Algorithms and Data Structures
- Graph Algorithms: Algorithms for traversing and manipulating graphs, such as Dijkstra's and A* for the shortest path, Kruskal’s and Prim’s for minimum spanning trees, and various algorithms for network flow.
- Dynamic Programming: A method for solving complex problems by breaking them down into simpler subproblems, and storing the results of subproblems to avoid redundant computations.
- Advanced-Data Structures: Structures like balanced trees (e.g., AVL trees, Red-Black trees), B-trees for databases, Tries for efficient retrieval, and Hash Maps for average constant-time complexity operations.
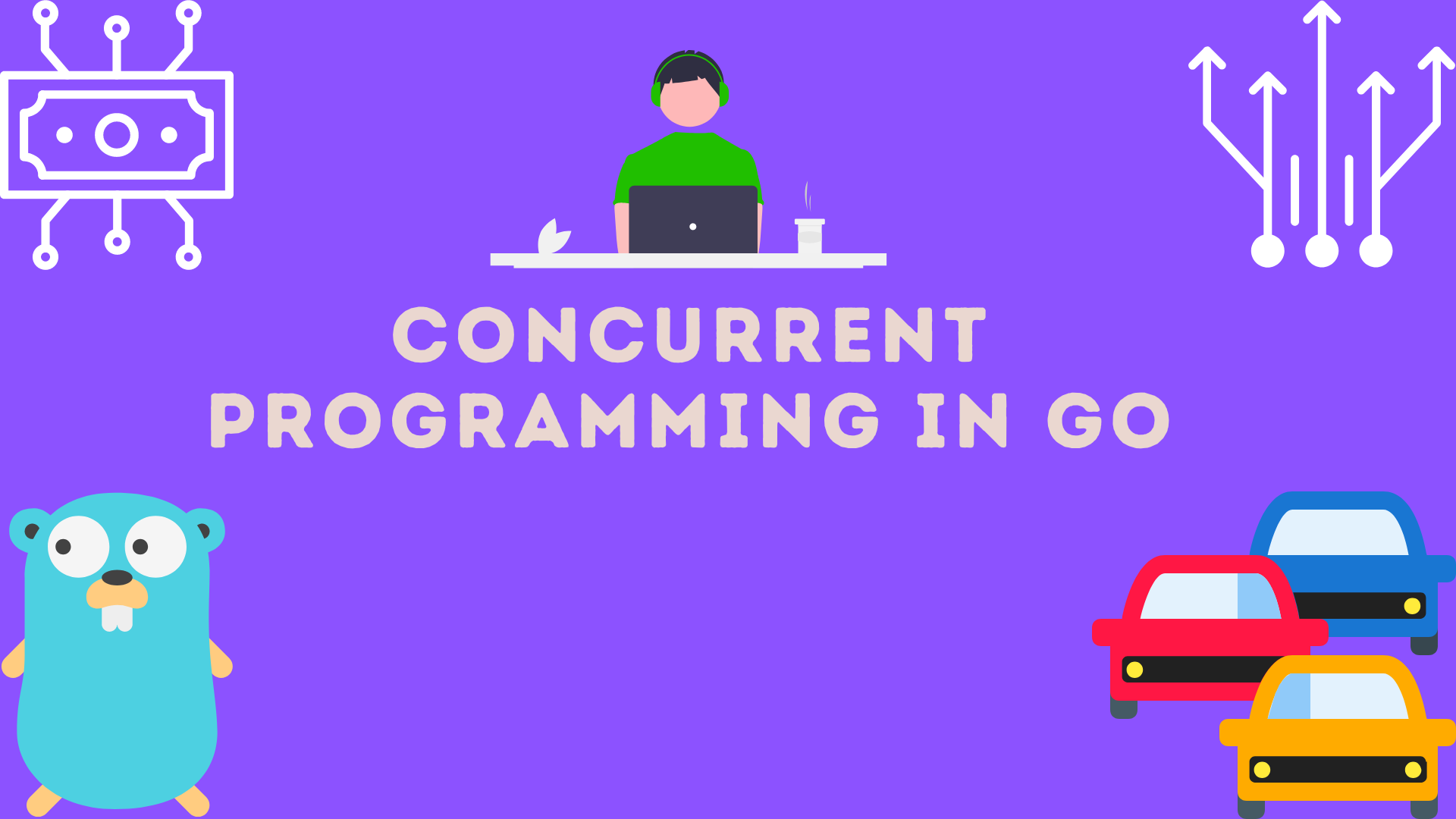
2. Concurrent and Parallel Programming
- Multithreading: Techniques to allow multiple threads to execute simultaneously, which can improve the efficiency of a program, particularly on multi-core processors. This includes understanding thread lifecycle, synchronization, deadlock prevention, and concurrent data structures.
- Parallel Algorithms: Algorithms designed to be executed simultaneously across multiple processors or cores. This includes divide-and-conquer strategies, parallel sorting, and map-reduce paradigms.
- Asynchronous Programming: Programming that allows processes to run independently of the main application flow, which can improve performance in I/O-bound and high-latency operations. This involves futures, promises, async/await constructs, and non-blocking I/O.
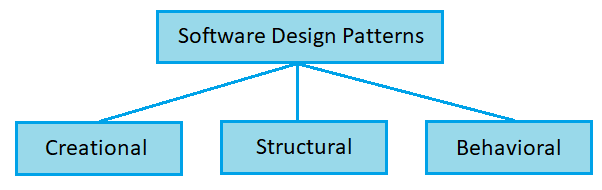
3. Software Design Patterns
- Creational Patterns: Methods to instantiate objects that promote code reuse and flexibility. Examples include Singleton, Factory, Abstract Factory, and Builder patterns.
- Structural Patterns: Ways to compose objects into larger structures while keeping these structures flexible and efficient. Examples include Adapter, Composite, Proxy, and Decorator patterns.
- Behavioural Patterns: Patterns that deal with object interaction and responsibility. Examples include Observer, Strategy, Command, and State patterns.
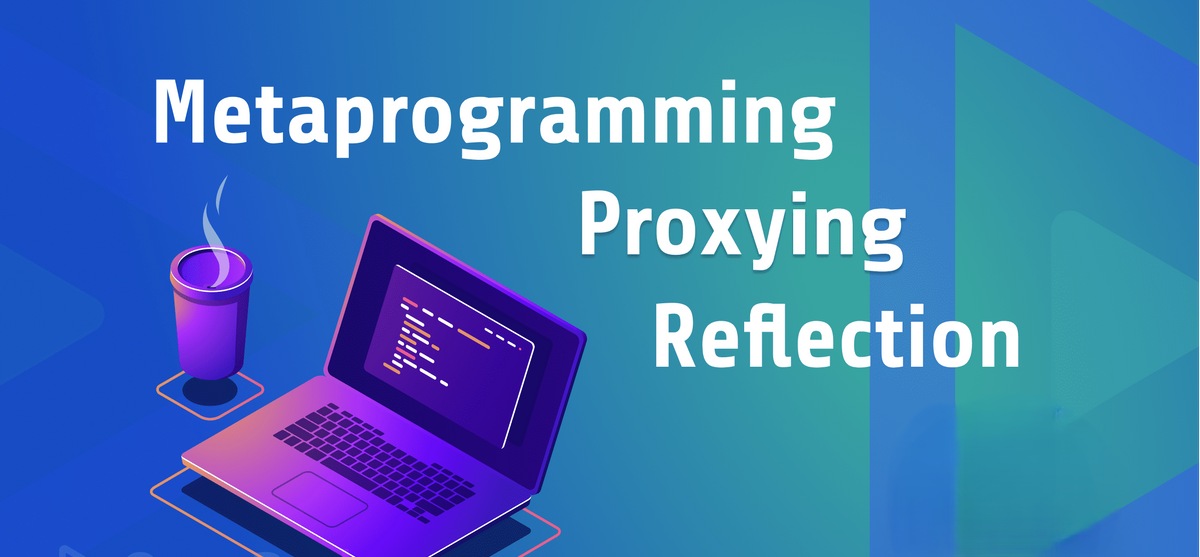
4. Metaprogramming and Reflection
- Metaprogramming: Writing programs that can manipulate themselves or other programs as their data. This includes techniques such as code generation, templates in C++, macros in Lisp, and annotations in Java.
- Reflection: The ability of a program to inspect and modify its structure and behavior at runtime. This is commonly used for dynamic type creation, serialization, and in frameworks that require runtime type information.
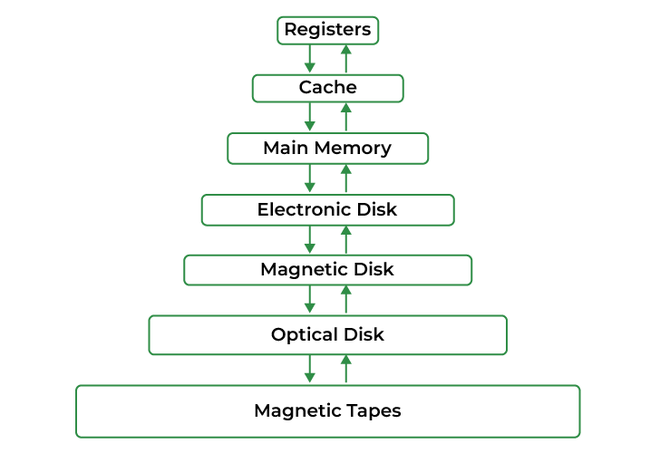
5. Memory Management and Optimization
- Garbage Collection: Techniques for automatic memory management involve reclaiming memory occupied by objects no longer in use. Different strategies include mark-and-sweep, generational garbage collection, and reference counting.
- Manual Memory Management: This is particularly relevant in languages like C and C++, where programmers must explicitly allocate and deallocate memory, requiring a deep understanding of pointers, memory leaks, and buffer overflows.
- Performance Tuning: Optimization techniques to improve code efficiency, including algorithmic optimizations, code profiling, and low-level optimizations such as loop unrolling and inlining functions.
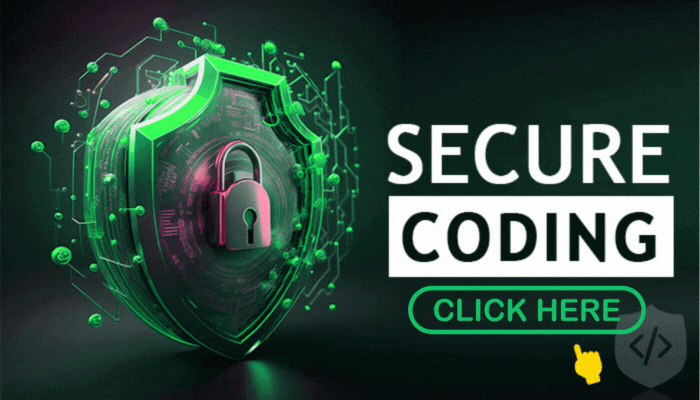
6. Secure Coding Practices
- Input Validation: Ensuring that all input to a program is properly checked and sanitized to prevent vulnerabilities such as SQL injection, cross-site scripting (XSS), and buffer overflow attacks.
- Cryptography: Implementing secure algorithms for data encryption, hashing, and communication protocols to protect data integrity and confidentiality.
- Access Control: Implementing robust mechanisms for authentication, authorization, and auditing to secure applications from unauthorized access.
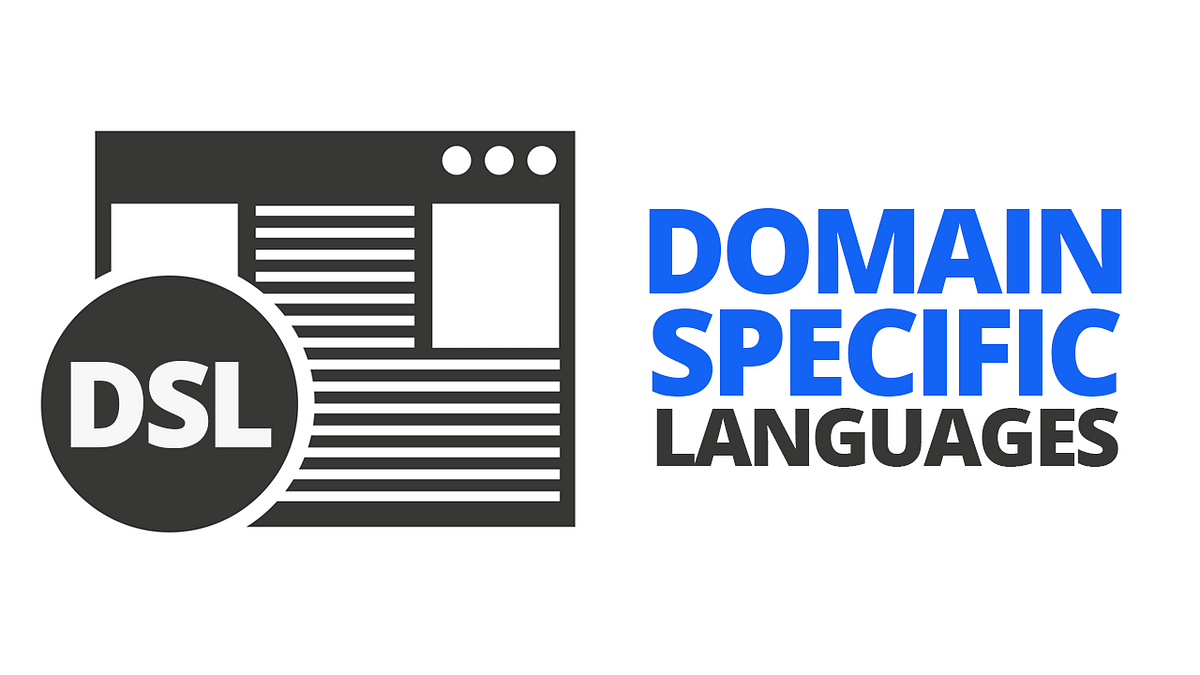
7. Domain-Specific Languages (DSLs)
- Design and Implementation: Creating languages tailored to specific aspects of a software system. Examples include SQL for database querying, HTML/CSS for web design, and VHDL for hardware description.
- Compiler Construction: Understanding the principles of designing and implementing compilers for these languages, including lexical analysis, parsing, semantic analysis, optimization, and code generation.
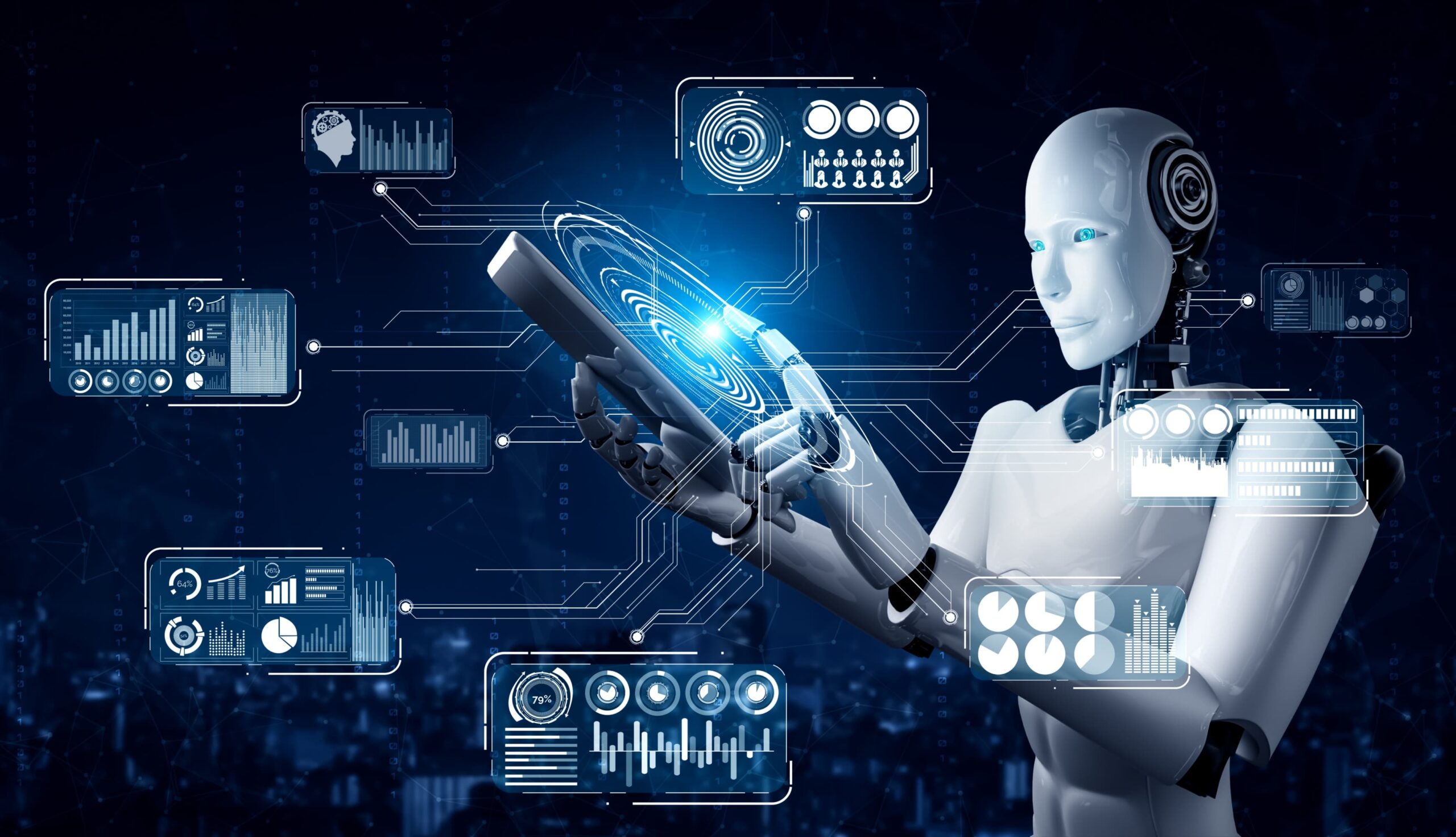
8. Machine Learning and AI Integration
- Algorithm Design: Implementing and optimizing machine learning algorithms such as decision trees, neural networks, support vector machines, and ensemble methods.
- Framework Utilization: Using advanced frameworks like TensorFlow, PyTorch, and Scikit-Learn to build, train, and deploy machine learning models.
- Data Engineering: Managing and preprocessing large datasets, feature engineering, and ensuring data quality for robust AI solutions.
Conclusion
Advanced coding encompasses a wide range of techniques and knowledge areas that are essential for building efficient, robust, and scalable software systems. Mastery of these advanced topics requires continuous learning and practice, as well as an understanding of both theoretical and practical aspects of software development.